Cancellation Offers in Spike
Currently only available for Stripe payment provider.
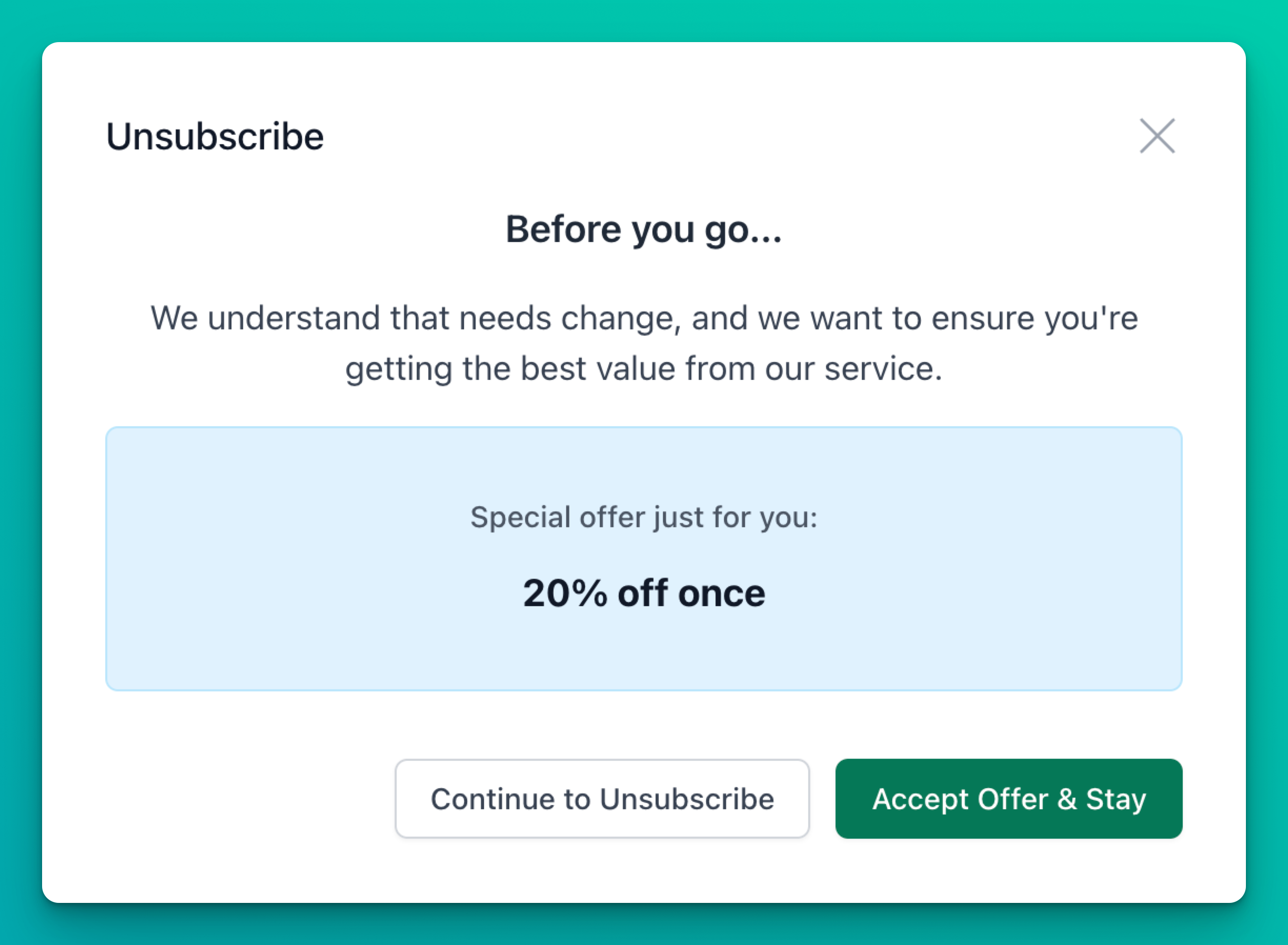
Introduction
Cancellation offers provide a way to retain users who are attempting to cancel their subscriptions by presenting them with special incentives to stay. Instead of immediately letting users cancel, you can configure a sequence of offers that will be shown to them during the cancellation process.
These offers could include discounts, extended trial periods, or other incentives that might convince the user to maintain their subscription. This strategy has been proven to significantly improve user retention in subscription-based services.
Simple Setup Using Configuration File
The simplest way to set up cancellation offers is through the configuration file. Add your offers to the spike.php
config file:
// config/spike.php
'stripe' => [
// ... other stripe config
'cancellation_offers' => [
new \Opcodes\Spike\Stripe\CouponCodeOffer(
couponId: 'discount_30_percent',
name: '30% off for 3 months',
description: 'Stay with us and get 30% off your subscription for the next 3 months.'
),
new \Opcodes\Spike\Stripe\PromotionCodeOffer(
promoCodeId: 'promo_yearly_deal',
name: 'Switch to yearly and save 20%',
description: 'Get 20% off when you switch to our annual plan.'
),
],
],
Each offer has the following constructor parameters:
couponId
/promoCodeId
: The Stripe ID of the coupon or promotion codename
: A short, attractive title for the offer (if not provided, will use the coupon name from Stripe)description
: A longer explanation of the benefit (optional)
The offers will be presented in the order they're defined in the config. When a user clicks to cancel, they'll first see the first offer. If they decline, they'll see the second offer, and so on.
When using the in-built Stripe Coupon/Promotion-Code offers, a subscription can only have one active. So, accepting a different coupon offer will replace any existing coupon on the subscription.
It's worth noting that for any custom offer types you may create, Spike does not keep track of which offers have been accepted, so it's possible for user to accept the same offer multiple times.
Advanced Setup Using Custom Resolver
For more complex scenarios, you can use a custom resolver to dynamically determine which offers to show based on user characteristics or behavior. Register your custom resolver in a service provider:
// AppServiceProvider.php
public function boot()
{
Spike::resolveCancellationOffersUsing(function ($billable, $defaultOffers) {
// Check if this is a premium user
if ($billable->isPremium()) {
// Premium users get a better offer
return collect([
new \Opcodes\Spike\Stripe\CouponCodeOffer(
'premium_discount_50_percent',
'Exclusive: 50% off for 6 months',
'As a valued premium customer, we\'d like to offer you 50% off for the next 6 months.'
),
]);
}
// Users with high usage get a special offer
if ($billable->hasHighUsage()) {
// Add a custom offer to the default ones
return $defaultOffers->push(
new \Opcodes\Spike\Stripe\CouponCodeOffer(
'high_usage_reward',
'Power User Discount: 40% off',
'We noticed you\'re one of our power users. Stay with us and get 40% off!'
)
);
}
// Otherwise, just use the default offers from config
return $defaultOffers;
});
}
The resolver receives two parameters:
$billable
: The billable entity (user or team)$defaultOffers
: A collection of the default offers from the config file
You can completely replace the default offers, modify them, or return them as-is based on your logic.
Available Cancellation Offer Types
CouponCodeOffer
Applies a Stripe coupon code to the user's subscription.
new \Opcodes\Spike\Stripe\CouponCodeOffer(
couponId: 'stripe_coupon_id', // Stripe coupon ID
name: 'Offer name', // Display name (optional)
description: 'Description' // Longer description (optional)
)
PromotionCodeOffer
Applies a Stripe promotion code to the user's subscription.
new \Opcodes\Spike\Stripe\PromotionCodeOffer(
promoCodeId: 'stripe_promo_id', // Stripe promotion code ID
name: 'Offer name', // Display name (optional)
description: 'Description' // Longer description (optional)
)
The difference between CouponCodeOffer and PromotionCodeOffer is that promotion codes in Stripe can have additional restrictions (like usage limits or expiration dates) on top of the underlying coupon.
Creating Custom Cancellation Offer Types
You can create custom offer types by implementing the Offer
interface:
namespace Opcodes\Spike\Contracts;
interface Offer
{
public static function __set_state(array $data): Offer;
public function identifier(): string;
public function name(): string;
public function description(): string;
public function view(): ?string;
public function isAvailableFor(SubscriptionPlan $plan, mixed $billable): bool;
public function apply(mixed $billable): void;
}
Here's what each method does:
__set_state()
: Required for Laravel to cache the config. See the existing implementations for examples.identifier()
: A unique identifier for this offer.name()
: A short, appealing title for the offer.description()
: A longer explanation of the benefit (can be empty).view()
: The Blade view to use for rendering this offer (defaults to'spike::components.shared.offer-default'
).isAvailableFor()
: Determines if this offer is valid for the given subscription plan and billable.apply()
: Applies the offer to the billable entity.
Here's an example of a custom offer that extends the subscription term instead of providing a discount:
use Opcodes\Spike\Contracts\Offer;
use Opcodes\Spike\SubscriptionPlan;
class ExtendedTermOffer implements Offer
{
public function __construct(
protected int $extraDays,
protected ?string $name = null,
protected ?string $description = null,
) {
}
public static function __set_state(array $data): Offer
{
return new self(
$data['extraDays'],
$data['name'],
$data['description'],
);
}
public function identifier(): string
{
return 'extend_' . $this->extraDays . '_days';
}
public function name(): string
{
return $this->name ?? $this->extraDays . ' days extra free';
}
public function description(): string
{
return $this->description ?? '';
}
public function view(): ?string
{
return 'spike::components.shared.offer-default';
}
public function isAvailableFor(SubscriptionPlan $plan, mixed $billable): bool
{
// Only available for paid plans
return $plan->isPaid();
}
public function apply(mixed $billable): void
{
// Extend the current subscription
$subscription = \Opcodes\Spike\Facades\PaymentGateway::billable($billable)
->getSubscription();
if ($subscription) {
$subscription->extendTrial($this->extraDays);
}
}
}
You can then use this custom offer in your config or resolver just like the built-in offer types.
It's worth noting that Spike does not keep track of which offers have been accepted, so it's possible for user to accept the same offer multiple times. Make sure to cover this in your isAvailableFor()
method logic, so the user does not get presented with the same offer again once they've already accepted it. Or, alternatively, make sure the apply
method does not apply the benefit again if it has already been applied before. These decisions are up to you, but it's worth knowing the limitations.